Python Pre API¶
Learn by example – Examples
Python training videos – Python, API, Scripting
Detailed Windows Setup guide – Windows Python Setup Guide, or Environment Setup for Windows with VS Code
Overview¶
The Python pre-processing API allows you to create and interact with M-Star models (*.msb files) from Python. The M-Star model is assembled from a few basic types: MStarModel, MStarComponent, and IProperty.
MStarModel
Corrosponds to a specific msb file. Contains any number of
MStarComponent
objects.MStarComponent
Each object in the model such as a static, moving body, scalar field, etc. Contains any number of
IProperty
objects. Some objects may support additional interfaces.IProperty
Each input on a property grid is defined. Each IProperty has a Name and a Value. The value may be a on/off switch, choice, text, floating point values, points, or other type. The property Category may be used to access specific properties.
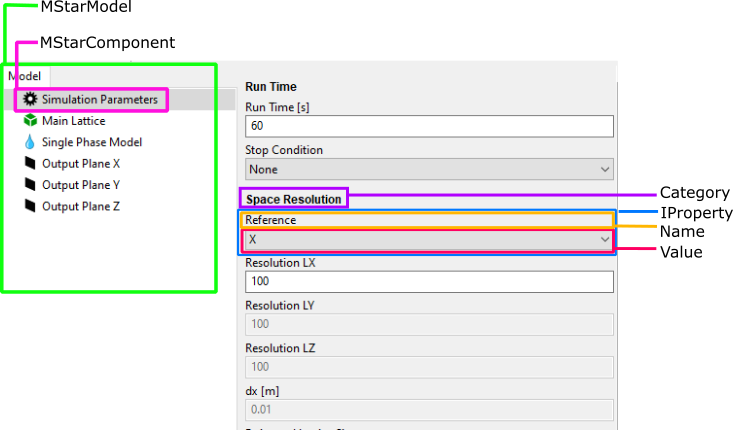
Example -
import mstar
mstar.CheckOutLicense()
# Create a new empty Model
model = mstar.Load()
# Get a Component, in this case the simulation parameters
sp = model.GetSimParams()
# Write out an html file of all the available properties
model.DumpHtmlFile("model.html")
# Change the 'Reference' and 'Resolution LY' properties on the simulation parameters
sp.Get("Space Resolution", "Reference").Value = "Y"
sp.Get("Space Resolution", "Resolution LY").Value = 120
# Because the name 'Resolution LY' is unique in the object, you can also access it with the Get method
sp.Get("Resolution LY").Value = 120
# Add a particles component to the model
particles = model.AddComponent("Particles")
# Change a property value using the category and name
particles.Get("Break-Up/Coalesce", "Breakup Enabled").Value = True
After you work with the model, you will typically save, export or possibly even run the solver directly from your python code. Some example scripts are provided in this documentation.